In this tutorial, we'll learn about the problems with styling React applications and how we can solve them using Styletron.
Introduction
I've been using React for quite some time now. In that time, the one thing which I've felt is most difficult while creating React applications is how to style them in a maintainable manner. There are various ways in which we can styles applications:
All of these will generate classes based on how we write the styles for each class.
const Button = styled.button`
background: ${(props) => (props.primary ? "palevioletred" : "white")};
color: ${(props) => (props.primary ? "white" : "palevioletred")};
font-size: 1em;
margin: 1em;
padding: 0.25em 1em;
border: 2px solid palevioletred;
border-radius: 3px;
`;
render(
<div>
<Button>Normal</Button>
<Button primary>Primary</Button>
</div>,
);
The above code will generate the following HTML:
<div>
<button class="sc-fzXfMz bXoZBN">Normal</button>
<button class="sc-fzXfMz fLZIOt">Primary</button>
</div>
It will also generate the following buttons:
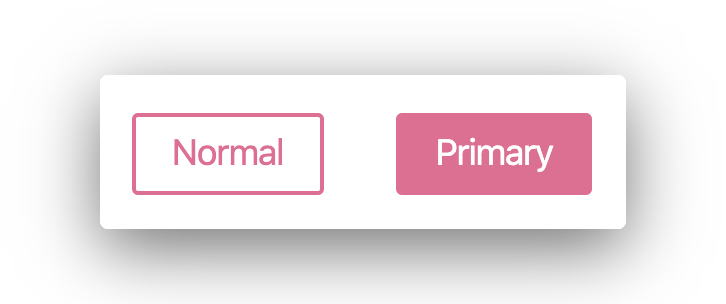
The generate CSS will be:
.bXoZBN {
color: palevioletred;
font-size: 1em;
background: white;
margin: 1em;
padding: 0.25em 1em;
border-width: 2px;
border-style: solid;
border-color: palevioletred;
border-image: initial;
border-radius: 3px;
}
.fLZIOt {
color: white;
font-size: 1em;
background: palevioletred;
margin: 1em;
padding: 0.25em 1em;
border-width: 2px;
border-style: solid;
border-color: palevioletred;
border-image: initial;
border-radius: 3px;
}
As we can see here that based on the styles, each component will generate a different class. This is very good when we want to create a component library or we want to create separate styles for everything in our application. The downside is that, as our application grows, the amount of styles also grows. In most cases, these styles are injected inline and are downloaded before the HTML is rendered on the page. Having a large amount of inline-styles will slow down the initial loading of your page. However, once the page is cached, the subsequent page loads will be faster.
What if the CSS-in-JS adapter we're using could generate classes for each style definition and then re-use them across all our components. For example, if our adapter could generate a class like:
.text-white {
color: white;
}
Then, this could be re-used in our component to generate HTML like:
<div>
<button class="text-white bg-orange">Normal</button>
<button class="text-white bg-red">Primary</button>
</div>
In this case, the amount of styles will be much lesser as the adapter isn't creating new styles for all the components. Instead, it's creating classes for each style definition and re-using them for all our components.
This is exactly what Styletron does.
We can just code like:
import { useStyletron } from "styletron-react";
export default () => {
const [css] = useStyletron();
return (
<a
href="/getting-started"
className={css({
fontSize: "20px",
color: "red",
})}
>
Start!
</a>
);
};
The above code will generate the following HTML:
<html>
<head>
<style>
.foo {
font-size: 20px;
}
.bar {
color: red;
}
</style>
</head>
<body>
<a href="/getting-started" class="foo bar">Start!</a>
</body>
</html>
It will also generate the following link:
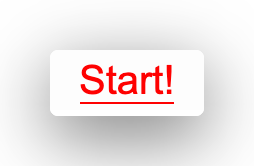
Features of Styletron
Styletron has a host of features like:
- Defining our own Media Queries And Pseudo Classes
- Using CSS Selectors
- Using our own Fonts
- Using Keyframes
- Vendor Prefixes are added by Styletron
- Theming
- Testing
- Debugging
Usages
We can use Styletron with React. It already has examples for using with the following:
Conclusion
In this article, we've learnt about Styletron and how it can help us reduce the amount of styles that we ship with our application. I hope that this article helps you in your future projects.